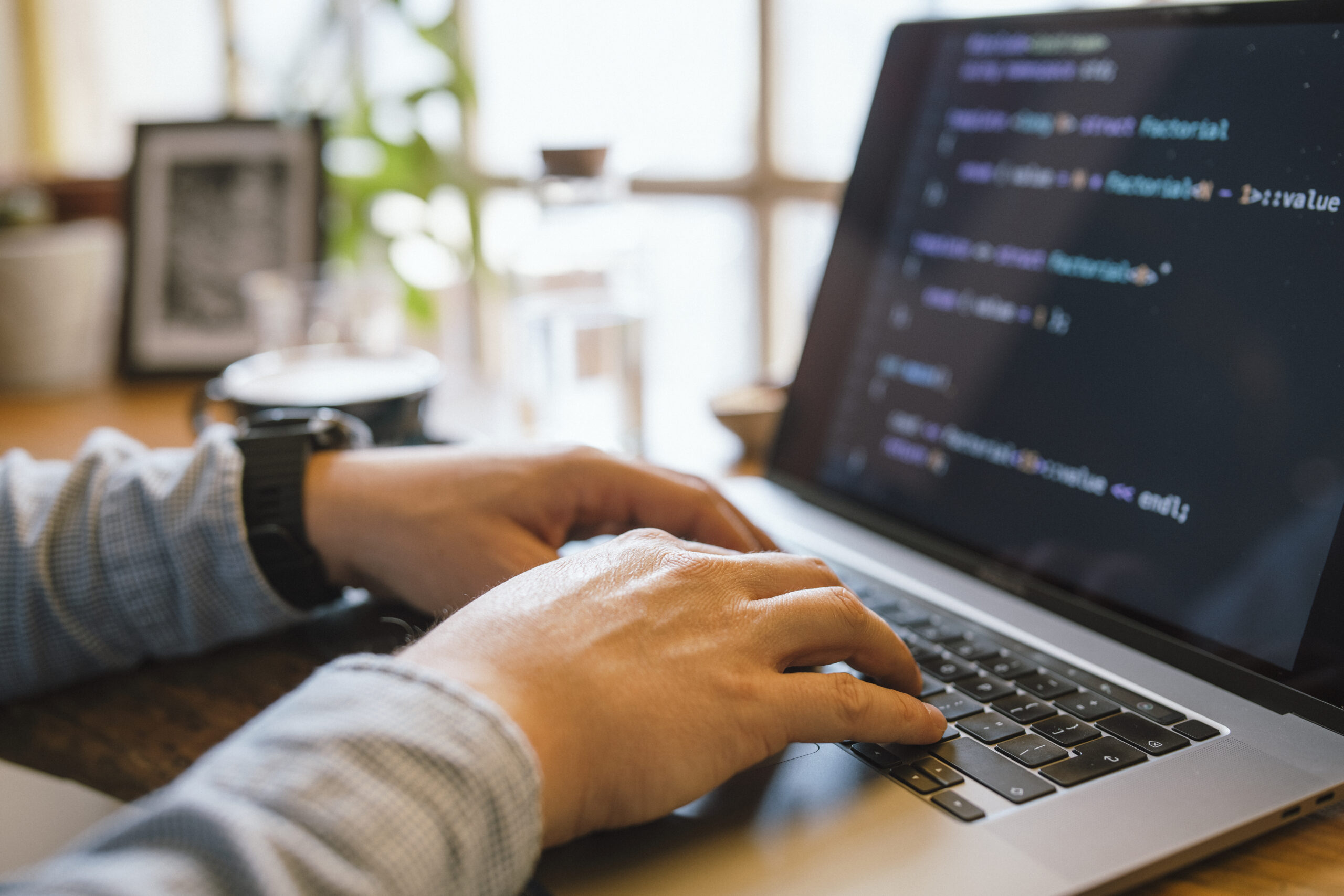
Debugging is Probably the most vital — yet frequently disregarded — techniques inside of a developer’s toolkit. It's not just about fixing broken code; it’s about comprehending how and why items go Improper, and Finding out to Consider methodically to resolve challenges successfully. Irrespective of whether you are a starter or simply a seasoned developer, sharpening your debugging competencies can help you save several hours of annoyance and radically help your efficiency. Here's many approaches to help you developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest methods builders can elevate their debugging techniques is by mastering the instruments they use every single day. Although creating code is one Element of progress, being aware of the best way to interact with it correctly for the duration of execution is equally vital. Modern-day advancement environments come Geared up with effective debugging capabilities — but quite a few developers only scratch the area of what these equipment can do.
Acquire, as an example, an Built-in Growth Atmosphere (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools allow you to established breakpoints, inspect the worth of variables at runtime, stage via code line by line, as well as modify code over the fly. When applied appropriately, they let you notice just how your code behaves throughout execution, which happens to be priceless for tracking down elusive bugs.
Browser developer applications, for example Chrome DevTools, are indispensable for front-close builders. They permit you to inspect the DOM, observe network requests, view true-time overall performance metrics, and debug JavaScript in the browser. Mastering the console, resources, and network tabs can turn annoying UI problems into workable tasks.
For backend or program-amount developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB provide deep Regulate more than working procedures and memory management. Mastering these tools might have a steeper Mastering curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, come to be comfy with Edition Regulate units like Git to know code historical past, uncover the precise instant bugs were being released, and isolate problematic changes.
In the end, mastering your equipment signifies heading outside of default configurations and shortcuts — it’s about acquiring an personal knowledge of your development atmosphere in order that when concerns come up, you’re not misplaced at nighttime. The higher you understand your equipment, the more time you'll be able to devote solving the actual problem instead of fumbling through the procedure.
Reproduce the condition
One of the more significant — and infrequently neglected — methods in successful debugging is reproducing the trouble. Prior to jumping into your code or building guesses, developers require to create a dependable natural environment or circumstance exactly where the bug reliably seems. Devoid of reproducibility, repairing a bug results in being a video game of likelihood, frequently bringing about squandered time and fragile code adjustments.
The first step in reproducing a challenge is collecting just as much context as is possible. Request questions like: What steps led to The difficulty? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you have got, the much easier it gets to be to isolate the precise situations less than which the bug happens.
Once you’ve gathered enough facts, attempt to recreate the situation in your local natural environment. This could signify inputting exactly the same facts, simulating comparable consumer interactions, or mimicking system states. If The problem seems intermittently, think about producing automatic exams that replicate the sting cases or state transitions included. These checks not just enable expose the problem but in addition reduce regressions in the future.
Often, The difficulty might be setting-unique — it might take place only on selected functioning methods, browsers, or beneath unique configurations. Applying resources like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating such bugs.
Reproducing the issue isn’t only a step — it’s a state of mind. It needs endurance, observation, and also a methodical solution. But once you can regularly recreate the bug, you are presently halfway to repairing it. Using a reproducible circumstance, You should utilize your debugging instruments additional correctly, exam opportunity fixes properly, and connect extra Evidently with all your workforce or buyers. It turns an summary criticism right into a concrete problem — and that’s exactly where developers prosper.
Examine and Fully grasp the Mistake Messages
Error messages tend to be the most respected clues a developer has when some thing goes wrong. Rather than looking at them as discouraging interruptions, builders must discover to take care of mistake messages as direct communications in the system. They normally inform you just what happened, where by it took place, and at times even why it happened — if you know the way to interpret them.
Start off by reading through the message thoroughly and in full. Quite a few developers, specially when underneath time stress, look at the primary line and instantly get started generating assumptions. But deeper from the error stack or logs may perhaps lie the correct root cause. Don’t just duplicate and paste error messages into search engines like google and yahoo — read and recognize them initial.
Crack the error down into sections. Is it a syntax mistake, a runtime exception, or a logic error? Will it stage to a selected file and line quantity? What module or purpose triggered it? These issues can manual your investigation and position you towards the accountable code.
It’s also handy to know the terminology with the programming language or framework you’re utilizing. Error messages in languages like Python, JavaScript, or Java normally stick to predictable designs, and learning to recognize these can considerably speed up your debugging method.
Some glitches are vague or generic, and in People conditions, it’s essential to examine the context where the mistake occurred. Examine linked log entries, enter values, and recent adjustments while in the codebase.
Don’t ignore compiler or linter warnings either. These frequently precede more substantial difficulties and supply hints about potential bugs.
In the end, error messages will not be your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a additional economical and self-assured developer.
Use Logging Wisely
Logging is Probably the most effective equipment within a developer’s debugging toolkit. When utilised properly, it offers true-time insights into how an software behaves, supporting you recognize what’s occurring beneath the hood with no need to pause execution or stage with the code line by line.
An excellent logging method starts with understanding what to log and at what level. Common logging levels involve DEBUG, INFO, WARN, Mistake, and Deadly. Use DEBUG for thorough diagnostic data in the course of improvement, INFO for general situations (like prosperous start out-ups), WARN for possible challenges that don’t crack the appliance, ERROR for precise challenges, and Deadly when the procedure can’t keep on.
Stay away from flooding your logs with excessive or irrelevant details. Excessive logging can obscure crucial messages and slow down your process. Give attention to important situations, condition modifications, enter/output values, and demanding decision factors inside your code.
Structure your log messages Obviously and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s simpler to trace problems in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs let you observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all with out halting This system. They’re especially worthwhile in production environments the place stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about balance and clarity. By using a well-believed-out logging tactic, you can decrease the time it will require to identify difficulties, obtain further visibility into your programs, and improve the Over-all maintainability and dependability within your code.
Think Just like a Detective
Debugging is not simply a technological task—it's a kind of investigation. To proficiently detect and repair bugs, developers need to strategy the method just like a detective resolving a secret. This mindset assists break down sophisticated difficulties into workable pieces and abide by clues logically to uncover the root cause.
Begin by here gathering evidence. Look at the signs of the challenge: mistake messages, incorrect output, or effectiveness difficulties. Much like a detective surveys against the law scene, acquire as much suitable facts as you could without the need of leaping to conclusions. Use logs, take a look at scenarios, and consumer studies to piece collectively a clear image of what’s happening.
Next, form hypotheses. Ask you: What can be producing this habits? Have any alterations just lately been created towards the codebase? Has this problem happened right before underneath equivalent situations? The goal should be to slender down opportunities and recognize potential culprits.
Then, exam your theories systematically. Endeavor to recreate the trouble inside a managed setting. Should you suspect a specific purpose or element, isolate it and confirm if the issue persists. Like a detective conducting interviews, talk to your code inquiries and Allow the effects direct you closer to the reality.
Shell out close notice to modest particulars. Bugs frequently cover inside the least envisioned areas—similar to a missing semicolon, an off-by-a person error, or a race affliction. Be thorough and client, resisting the urge to patch the issue devoid of totally knowledge it. Short-term fixes may well hide the true problem, only for it to resurface later on.
Last of all, preserve notes on Anything you attempted and figured out. Just as detectives log their investigations, documenting your debugging method can help you save time for long term troubles and help Other individuals have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical skills, method troubles methodically, and come to be more effective at uncovering hidden troubles in advanced systems.
Compose Assessments
Producing checks is one of the most effective approaches to transform your debugging competencies and overall improvement effectiveness. Exams not merely enable capture bugs early but will also function a security Web that provides you assurance when creating alterations on your codebase. A nicely-tested application is easier to debug mainly because it allows you to pinpoint exactly exactly where and when a problem occurs.
Start with device checks, which deal with unique capabilities or modules. These smaller, isolated assessments can promptly expose no matter whether a certain piece of logic is Functioning as expected. When a test fails, you straight away know where by to glimpse, appreciably cutting down time invested debugging. Unit checks are Specially valuable for catching regression bugs—concerns that reappear following previously remaining fastened.
Up coming, integrate integration checks and conclude-to-stop tests into your workflow. These enable be certain that different parts of your software perform together effortlessly. They’re notably helpful for catching bugs that manifest in intricate methods with various parts or solutions interacting. If a little something breaks, your exams can show you which Section of the pipeline failed and beneath what circumstances.
Producing exams also forces you to definitely Consider critically about your code. To test a element effectively, you would like to grasp its inputs, expected outputs, and edge situations. This level of comprehension Normally sales opportunities to better code construction and much less bugs.
When debugging an issue, composing a failing test that reproduces the bug could be a robust first step. After the exam fails regularly, it is possible to focus on repairing the bug and enjoy your test move when The problem is fixed. This method makes sure that a similar bug doesn’t return in the future.
In a nutshell, producing checks turns debugging from a aggravating guessing match right into a structured and predictable process—aiding you catch additional bugs, a lot quicker and much more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to become immersed in the issue—looking at your display for hrs, hoping Option just after solution. But Probably the most underrated debugging equipment is just stepping away. Using breaks aids you reset your brain, lessen annoyance, and infrequently see The problem from the new point of view.
When you are also near to the code for also extended, cognitive tiredness sets in. You could commence overlooking apparent mistakes or misreading code that you simply wrote just hours earlier. During this point out, your Mind gets considerably less productive at issue-solving. A brief stroll, a coffee break, or even switching to another undertaking for 10–15 minutes can refresh your focus. Lots of builders report obtaining the root of a problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, Primarily through more time debugging sessions. Sitting down in front of a monitor, mentally caught, is not just unproductive but will also draining. Stepping absent enables you to return with renewed Electrical power and also a clearer attitude. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
When you’re stuck, a very good guideline should be to set a timer—debug actively for forty five–60 minutes, then have a 5–10 moment break. Use that point to move all around, extend, or do anything unrelated to code. It may well experience counterintuitive, Specifically less than restricted deadlines, but it really truly causes quicker and more practical debugging in the long run.
In a nutshell, having breaks just isn't an indication of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and aids you steer clear of the tunnel eyesight that often blocks your progress. Debugging is often a psychological puzzle, and rest is a component of fixing it.
Master From Every Bug
Every single bug you come upon is more than just A brief setback—It can be a possibility to grow like a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can train you a thing important if you take some time to mirror and examine what went Erroneous.
Start out by inquiring you a few important queries after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater techniques like device screening, code testimonials, or logging? The solutions typically reveal blind spots inside your workflow or knowing and enable you to Construct more powerful coding routines shifting forward.
Documenting bugs can also be an excellent pattern. Continue to keep a developer journal or manage a log in which you Notice down bugs you’ve encountered, how you solved them, and Everything you learned. Over time, you’ll begin to see designs—recurring concerns or typical mistakes—that you can proactively stay clear of.
In staff environments, sharing Whatever you've discovered from a bug with the friends may be especially impressive. No matter if it’s by way of a Slack information, a brief create-up, or A fast expertise-sharing session, assisting others steer clear of the identical issue boosts staff efficiency and cultivates a much better Finding out culture.
Additional importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. Rather than dreading bugs, you’ll start out appreciating them as crucial aspects of your growth journey. After all, many of the very best builders aren't those who write best code, but those who repeatedly learn from their problems.
Eventually, Each and every bug you take care of adds a different layer to your ability established. So next time you squash a bug, take a minute to replicate—you’ll arrive away a smarter, extra capable developer on account of it.
Summary
Improving your debugging expertise usually takes time, practice, and persistence — although the payoff is large. It tends to make you a more successful, self-assured, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, remember: debugging isn’t a chore — it’s a chance to become greater at Anything you do.